C# .NET MVC实现图片、视频上传接口
|
admin
2025年1月3日 10:5
本文热度 3322
|
在程序开发项目中我们经常会上传图片或者视频,下面介绍一下用.NET MVC实现图片、视频上传接口的三种方式;有兴趣的小伙伴们可以一起学习。
一、 Base64图片上传
适合小程序端
/// </summary>
/// <param name="base64String"></param>
/// <param name="filePath"></param>
/// <param name="fileName"></param>
/// <returns></returns>
public static string UpLoadImage(string base64String)
{
FileStream fs = null;
BinaryWriter bw = null;
try
{
if (string.IsNullOrEmpty(base64String))
{
throw new Exception("图片参数不能为空");
}
string filePath = "/UpLoad/" + DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day;
string realPath = HttpContext.Current.Server.MapPath("~/" + filePath);
string day = YearMonthDay;
if (!Directory.Exists(realPath))//如果不存在就创建file文件夹
{
Directory.CreateDirectory(realPath);
}
string imagePath = filePath + "/" + fileName;
string imageSavePath = realPath + "/" + fileName;
byte[] arr = Convert.FromBase64String(base64String);
fs = new System.IO.FileStream(imageSavePath, System.IO.FileMode.Create);
bw = new System.IO.BinaryWriter(fs);
bw.Write(arr);
bw.Close();
fs.Close();
return imagePath;
}
catch (Exception ex)
{
if (bw != null)
{
bw.Close();
}
if (fs != null)
{
fs.Close();
}
throw new Exception(ex.Message);
}
}
二、HttpPostedFile文件上传
适合网页端
/// <summary>
/// 上传图片、视频 HttpPostedFile
/// </summary>
/// <returns></returns>
[HttpPost]
public ApiResponse UploadPostFile()
{
try
{
string filePath = "/UpLoad/" + DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day;
string realPath = HttpContext.Current.Server.MapPath("~/" + filePath);
if (!Directory.Exists(realPath))//如果不存在就创建file文件夹
{
Directory.CreateDirectory(realPath);
}
HttpPostedFile file = HttpContext.Current.Request.Files[0];
string fileType = HttpContext.Current.Request.Form["fileType"];
string imagePath = filePath + "/" + "test.jpg";
file.SaveAs(imagePath);
return imagePath;
}
catch (Exception e)
{
return BaseApiResponse.ApiError(e.Message);
}
}
三、FormData上传(通过表单(multipart/form-data)的方式上传后)
安装MultipartDataMediaFormatter
路由配置
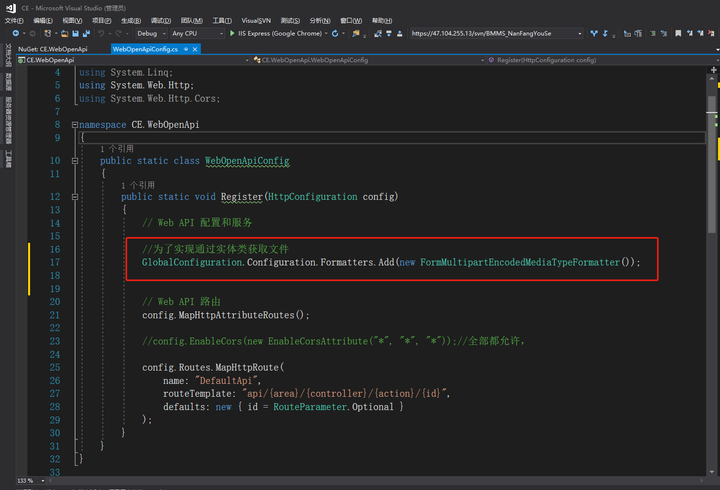
配置代码
GlobalConfiguration.Configuration.Formatters.Add(new FormMultipartEncodedMediaTypeFormatter());
file和表单一起
/// <param name="formData"></param>
/// <returns></returns>
[HttpPost]
public string UploadFormData(FormData formData)
{
try
{
string filePath = "/UpLoad/" + DateTime.Now.Year + "/" + DateTime.Now.Month + "/" + DateTime.Now.Day;
string realPath = HttpContext.Current.Server.MapPath("~/" + filePath);
if (!Directory.Exists(realPath))//如果不存在就创建file文件夹
{
Directory.CreateDirectory(realPath);
}
string fileType = formData.Fields[0].Value;
string imagePath = filePath + "/" + "test.jpg";
FileStream fs = null;
BinaryWriter bw = null;
byte[] arr = formData.Files[0].Value.Buffer;
fs = new System.IO.FileStream(imagePath, System.IO.FileMode.Create);
bw = new System.IO.BinaryWriter(fs);
bw.Write(arr);
bw.Close();
fs.Close();
return imagePath;
}
catch (Exception e)
{
throw new Exception(ex.Message);
}
}
四、用Postman测试一下吧
成功接收到图片
该文章在 2025/1/3 16:54:23 编辑过